Building a Real-time Chat Application with Wix Velo and Wix Real-time API
- Faysal Jaali
- Aug 15, 2023
- 8 min read
Updated: Feb 8, 2024
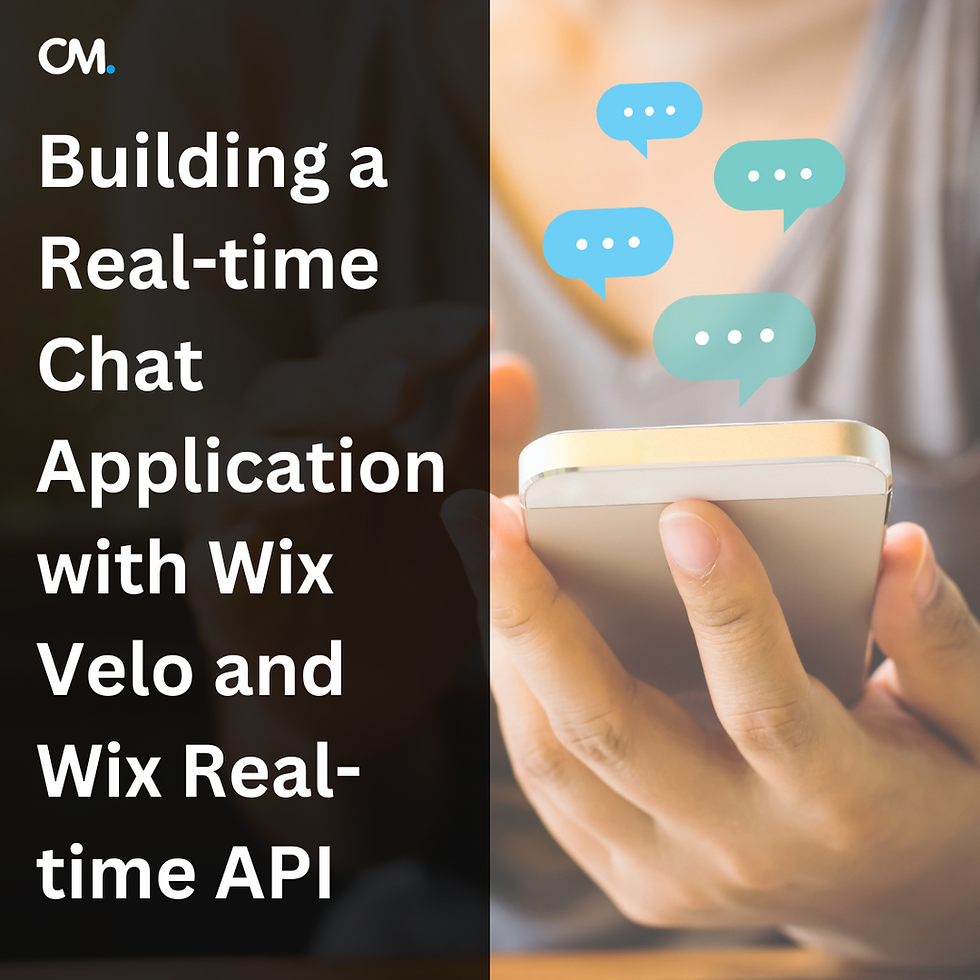
Real-time chat functionality has become an essential feature in many web applications today, be it e-commerce sites, social networking platforms, or collaborative tools. If you're working with Wix to create your website, there's good news: You can easily build a real-time chat application using Wix Velo and the Wix Real-time API.
In this blog, I'll guide you through the process of creating a chat application step-by-step.
Prerequisites:
A Wix account.
Basic understanding of JavaScript and Wix Velo.
Step 1: Set Up Your Wix Site
Before we begin, make sure you have a Wix site to work on. If you don’t have one yet, create it.
Step 2: Enable Velo Development Mode
Navigate to your site's editor.
Click on the Dev Mode on the top bar and turn it on.
This will unveil the Velo side panel which provides backend and frontend functionality.
Step 3: Create a Chat Collection
3.1. Accessing the Content Manager:
Navigation:
In your Wix Editor, locate the left sidebar.
Click on Database to access the Content Manager. If it's your first time accessing the database, you may need to accept some initial terms or introductory pop-ups.
Creating a New Collection: Once inside the Content Manager, click on + New Collection.
Naming the Collection: Give your new collection a name, for this tutorial, we'll name it Chat. This name will be used to reference the collection in our code.
3.2. Defining Fields for the Collection:
Once the collection is created, it's time to set up fields which will store specific pieces of data for each chat message.
Username Field:
Click on + Add Field.
Name the field username.
Set the field type to Text.
This field will store the name of the user sending the message.
Message Field:
Click on + Add Field again.
Name this field message.
Set its type to Text.
This is where the actual chat message will be stored.
Timestamp Field:
Click on + Add Field once more.
Name this field timestamp.
Set its type to Date and Time.
This will automatically store the date and time when each message is sent, enabling the messages to be displayed chronologically.
3.3. Setting Permissions for the Collection:
For a chat application, you'll want users to be able to add messages but not necessarily modify or delete them after they've been posted. Setting permissions ensures data integrity and security.
Setting Permissions: Inside the Chat collection, navigate to the Permissions section.
Read Permissions: Set this to "Anyone" because we want any user to be able to read the chat messages.
Create Permissions: Also set to "Anyone" since users should be able to create (or send) messages.
Update & Delete Permissions: Set these to "Admin" or "Site member author". This way, messages can only be modified or deleted by the site's administrators or the original authors, depending on your preference.
3.4. Testing the Collection:
Once your fields are set and permissions are in place, you may want to manually add a few test entries:
Adding Test Data:
In the Content Manager, select your Chat collection.
Click on + Add Row and fill in the username, message, and timestamp fields.
Add a few messages to simulate actual chat data.
With the Chat collection now set up, you have a foundation to store and retrieve real-time chat messages. The subsequent steps will tie this database into your site's frontend, creating an interactive chat experience for users.
Step 4: Design the Chat UI
4.1. Setting Up the Repeater for Displaying Messages:
Adding the Repeater:
In your Wix Editor, go to the Add panel (usually on the left sidebar).
Navigate to List & Grids.
Select and drag a repeater element to the desired location on your page.
Configuring the Repeater:
Adjust the layout, spacing, and design to match your website's aesthetic.
For our chat, we need to display each user's name and their respective message. Thus, in the repeater's item box, we’ll need two text elements.
4.2. Adding Text Elements inside the Repeater:
Username Text Element:
Drag a text element inside the repeater's item box.
Name it, for clarity, something like "usernameText".
Modify its design, size, and font to your liking.
Message Text Element:
Similarly, drag another text element for the message itself.
Position it below or next to the username text element, based on your design preference.
Name it "messageText".
4.3. Input Fields for Chat Interaction:
Username Input Box:
Go to the Add panel and under User Input, choose Text Input.
Drag it to a suitable location below the repeater.
This will be where users enter their name. Name this input box as usernameInput.
Optional: Add a placeholder text like "Enter your name" to guide users.
Message Input Box:
Just like the username input, drag another text input box for users to type their message.
Position it next to the username input or directly below it.
Name it messageInput and maybe set a placeholder like "Type your message...".
4.4. The Send Button:
Adding the Button:
From the Add panel, select Button.
Drag a button to a location near the message input box.
Name it sendButton.
Configuring the Button:
Adjust its size, color, and design to fit your chat interface.
Set the button text to something clear, like "Send" or "Submit".
4.5. Styling and Final Touches:
Consistency: Ensure that all elements have a consistent design. This includes font types, sizes, and colors.
Spacing: Properly space your elements so that they're not too cramped. This improves user experience.
Feedback: Consider adding tooltips or helper text to guide new users.
Mobile Responsiveness: Ensure that your chat UI looks good and is functional on both desktop and mobile views. Wix provides tools to adjust the design for different screen sizes.
With the above steps, you should have a well-designed and functional chat UI that's ready to be powered by real-time functionality in the subsequent steps. Remember, UI is subjective, so feel free to get creative and tweak the design to match your site's theme and your personal preference!
Step 5: Add Real-time Functionality
5.1. Importing Necessary Modules
First, at the top of your page's code (usually in the Site Code section), you need to import the required Wix modules.
import wixData from 'wix-data';
import { getRealtime } from 'wix-realtime';
5.2. Initializing the Real-time Functionality
Using the 'getRealtime()' function, you can establish a connection for real-time interactions:
const realtime = getRealtime();
5.3. Setting Up Listeners for Real-time Updates
Now, you need to set up a listener to watch for new messages being added to the Chat collection.
realtime.onItemInserted('Chat', (item) => {
// This function will execute whenever a new message is added
loadMessages();
});
This listener watches for any new items (messages, in our case) inserted into the Chat collection and calls the 'loadMessages()' function every time it detects a new message.
5.4. Fetching and Displaying Messages
The 'loadMessages()' function will fetch messages from the Chat collection and display them in the repeater.
function loadMessages() {
wixData.query('Chat')
.ascending('timestamp')
// To ensure messages are loaded chronologically
.limit(50)
// Adjust based on how many messages you want to display at once
.find()
.then((results) => {
$w('#repeater').data = results.items;
// Bind the results to the repeater
})
.catch((err) => {
console.error("Error fetching messages:", err);
});
}
5.5. Implementing the Send Functionality
Capturing Input and Sending Message:
To make the chat interactive, allow users to send messages by capturing the input from the input boxes and adding it to the Chat collection:
export function sendButton_click(event) {
const username = $w('#usernameInput').value;
const message = $w('#messageInput').value;
if(username && message) {
// Ensure both fields have content
const chatData = {
"username": username,
"message": message,
"timestamp": new Date()
};
wixData.insert('Chat', chatData)
.then(() => {
$w('#messageInput').value = '';
// Clear the message input box after sending
})
.catch((err) => {
console.error("Error sending message:", err);
});
}
}
Attaching the Send Function to the Button:
Ensure that the sendButton_click function is tied to the onClick event of the "Send" button. You can do this either through the properties panel in the Wix editor or by manually setting it in code.
5.6. Initializing the Chat
Finally, when the page loads, you'd want to display any existing messages:
$w.onReady(() => {
loadMessages();
});
Conclusion
By completing step 5, you have integrated real-time capabilities into your chat application. Now, when one user sends a message, every other user will immediately see the update without any need for manual refreshes. This real-time interactivity provides a seamless chatting experience, making your platform more engaging and responsive.
Step 6: Comprehensive Example & Running the Application
6.1. Bringing It All Together:
After following Steps 1 through 5, you have individual pieces of code that handle various functionalities of the chat application. Now, let's illustrate how you'd combine them for your chat page's backend (chat.jsw):
// Import necessary modules
import wixData from 'wix-data';
import { getRealtime } from 'wix-realtime';
const realtime = getRealtime();
// Function to load messages
function loadMessages() {
wixData.query('Chat')
.ascending('timestamp')
.limit(50)
.find()
.then((results) => {
$w('#repeater').data = results.items;
})
.catch((err) => {
console.error("Error fetching messages:", err);
});
}
// Listen for real-time updates
realtime.onItemInserted('Chat', (item) => {
loadMessages();
});
// Run the function on page load
$w.onReady(() => {
loadMessages();
});
On your chat page's frontend, attach event handlers to relevant elements:
// Function for sending messages
export function sendButton_click(event) {
const username = $w('#usernameInput').value;
const message = $w('#messageInput').value;
if(username && message) {
const chatData = {
"username": username,
"message": message,
"timestamp": new Date()
};
wixData.insert('Chat', chatData)
.then(() => {
$w('#messageInput').value = '';
})
.catch((err) => {
console.error("Error sending message:", err);
});
}
}
6.2. Testing the Application:
Running in the Wix Editor:
Save all your changes and preview your site within the Wix Editor.
Use the chat feature to send and receive messages, ensuring the real-time updates work.
Testing on Different Devices:
Publish your Wix site.
Access the chat page on various devices (desktop, mobile, tablet) to ensure responsiveness and functionality.
Multiple User Testing:
Ask a few colleagues or friends to join the chat at the same time. This will help in checking the real-time features and load times with multiple users.
6.3. Debugging & Refinement:
Console Errors: While testing, keep an eye on the browser's console for any JavaScript errors or warnings. These can give insights into any issues with the chat.
Feedback Collection: Encourage test users to provide feedback about their experience. This can help in identifying areas of improvement.
Optimizations: Based on user feedback and your observations, make necessary tweaks. Maybe the chat needs to load faster, or perhaps the user interface requires some adjustments.
Conclusion:
Building a chat application can initially seem like a daunting task, especially if you're diving into the realms of real-time communication and dynamic user interactions. However, platforms like Wix Velo, coupled with the power of the Wix Realtime API, simplify this process immensely, making it accessible even to those without an extensive background in software development.
The beauty of this approach lies in its adaptability. With the basics in place, you can further customize your chat application, integrate additional Wix services, or even link external tools and APIs. Whether you're looking to foster a community, provide customer support, or just create a space for users to interact, a chat application can be the cornerstone of engagement on your website.
While this guide provides a robust foundation for building a chat application, crafting a sophisticated and seamlessly functioning digital product often requires specialized expertise. If you're looking to elevate your chat app or any other web application to professional standards, why not consult with experts?
CodeMasters Agency specializes in delivering top-tier digital solutions tailored to your unique needs. Our team of seasoned developers and design experts can transform your vision into reality, ensuring an impeccable user experience and cutting-edge functionality.
Don't leave your project to chance. Trust the masters of the craft. Get in touch with CodeMasters Agency today and propel your web projects to unparalleled heights!